දැම් අපි බලමු මොකක්ද මේ inheritance ක්රියාවලිය කියල, Inheritance යනු යම් class එකකකින් සාදන ලද වස්තුන්(objects) වෙනත් class එකකට අයත් objects වල ගුණාංගයන්ද අයත් කර ගැනීමේදී සිදුකරන ක්රියාවලියයි. එම නිසා මෙහිදී අදාල ලක්ෂණ අත් කරගත් class එක සහ ලක්ෂණ අත් කරගැනීමට බඳුන් වූ class එක සඳහා පොදු ලක්ෂණ එකක් හෝ කිහිපයක් පවතිනවා. අදාල ලක්ෂණ අත් කරගත් class එක child class එක නොහොත් sub class එක ලෙසත් ලක්ෂණ අත් කරගැනීමට බඳුන් වූ class එක parent class එක නොහොත් super class එක ලෙසත් හැඳින්වෙනවා. මෙම ක්රියාවලියේදී extends නම් ජාවා keyword එක භාවිතා වනවා. යම් class එකක් Inherite කිරීමෙන් සාදන ලද නව class එක එහි parent class එකෙහි attributes සහ behaviour යනාදිය උකහා ගන්නා නිසා මෙහිදී ඉබේම software re-usability නම් සංකල්පයද ක්රියාත්මක වනවා. නමුත් inherite කිරීමෙන් සාදාගන්නා ලද නව class එකෙහි එහි parent class එකේ නොමැති attributes සහ behaviours අන්තර්ගත වීමට පුලුවන්.
ජාවා තුල inheritance සංකල්පය ක්රියාවට නැංවීමේදී දැන ගතයුතු කරුණු.
- ► අලුතින් සාදන සෑම class එකක්ම අනිවාර්යයෙන්ම වෙනත් class එකක් extend (inherit) කල යුතුය.
- ► extend කරන්නේ කුමන class එකක්ද යන්න විශේෂයෙන් සඳහන් කර නැති විට Object යන class එක inherit වීම සිදුවේ. Object යනු ජාවා class hierarchy එකේ උඩින්ම ඇති super class එකයි.
- ► inherit කිරීමකදී කිසිවිටෙකත් constructors උකහා ගැනීම සිදුනොවේ. සියලුම constructors එය define කරන ලද class එක සඳහා වීශේෂ වේ. (constructor පිලිබඳ අපි මීට පෙර පාඩමකින් සාකච්ඡා කර තිබේ එම පාඩම සඳහා මෙතන click කරන්න)
- ► යම්කිසි sub class එකක් construct වීමේදී එම class එකෙහි super class එකෙහි constructor එක මුලින්ම call වීම සිදුවේ.
- ► ජාවාහිදී ඕනෑම class එකකට තිබිය හැක්කේ එක් parent class එකක් පමණි. එනම් multiple inheritance සඳහා ජාවා සහය නොදක්වයි. එමනිසා multiple inheritance මගින් සිදුවන කාර්යය ජාවාහිදී සිදුකරන්නේ interfaces භාවිතයෙනි. අපි ඉදිරි පාඩමකින් ජාවා interfaces පිලිබඳ අධ්යයනය කරමු. ජාවා multiple inheritance සඳහා සහාය නොදැක්වුවද C++ වලදී නම් multiple inheritance සඳහා ඉඩකඩ සලසා තිබේ.
class SubClassName extends SuperClassNameදැන් අපි මෙම inheritance සංකල්පය හොඳින් වටහා ගැනීම සඳහා සරල ජාවා වැඩසටහනක් ලියමු.
එම ජාවා වැඩසටහන සඳහා අපි පහත hierarchy එක භාවිතා කරමු.
Cube.java
/** *class : Cube *Author : Kanishka Dilshan *Purpose: Demonstrate inheritance in Java *Blog : http://javaxclass.blogspot.com */ class Cube { protected int length; protected int width; protected int height; public Cube(int l,int w,int h){ length=l; width=w; height=h; } public void setLength(int l){ length=l; } public void setWidth(int w){ width=w; } public void setHeight(int h){ width=h; } public int getVolume(){ return(length*width*height); } public void showInfo(){ System.out.println("Length \t:"+this.length); System.out.println("Width \t:"+this.width); System.out.println("Height \t:"+this.height); System.out.println("Volume \t:"+getVolume()); } }
ColorCube.java
/** *class : ColorCube *Author : Kanishka Dilshan *Purpose: Demonstrate inheritance in Java *Blog : http://javaxclass.blogspot.com */ class ColorCube extends Cube { String color; public ColorCube(int l,int w,int h,String color){ //constructing the parent super(l,w,h); this.color=color; } public void setColor(String color){ this.color=color; } public void showInfo(){ super.showInfo(); System.out.println("Color \t:"+this.color); } }
InheritanceDemo.java
/** *class : InheritanceDemo *Author : Kanishka Dilshan *Purpose: Demonstrate inheritance in Java *Blog : http://javaxclass.blogspot.com */ class InheritanceDemo { public static void main(String args[]){ Cube cube1=new Cube(3,7,5); ColorCube ccb1=new ColorCube(2,9,4,"Blue"); cube1.showInfo(); System.out.println("_____________________________"); ccb1.showInfo(); System.out.println("\nMaking changes to cube objects...."); cube1.setWidth(8); ccb1.setColor("Yellow"); ccb1.setHeight(6); //this methods is inherited by the Cube class System.out.println("New info. of modified objects\n"); cube1.showInfo(); System.out.println("_____________________________"); ccb1.showInfo(); } }Output :
ඉහත ජාවා වැඩසටහන අධ්යයනයෙන් ඔබට inheritance පිලිබඳ තවදුරටත් වටහාගත හැකිවනු ඇත.එහි class variables සඳහා protected access එකක් ලබාදීමෙන් සිදුකරන්නේ එම data members සඳහා ප්රවේශවීම(access) අදාල class එක තුලදී සහ එහි sub classes වලට පමණක් සීමා කිරීමයි.
දැන් අපි Inheritance හිදී සිදුවන ක්රියාවලිය සියුම්ව වටහා ගැනීමට සුදුසු ජාවා වැඩසටහනක් අධ්යයනය කරමු.
InherianceStudy.java
/** *class : InherianceStudy *Author : Kanishka Dilshan *Purpose: Demonstrate inheritance in Java *Blog : http://javaxclass.blogspot.com */ class Parent { protected int i,j; public Parent(){ System.out.println("Using the default constructor of Parent class.."); i=0; j=0; } public Parent(int i,int j){ System.out.println("Using the integer constructor of Parent class.."); this.i=i; this.j=j; } public void showMessage(String msg){ System.out.println("A message from Parent class.."); System.out.println("Message : "+msg); } } class Child extends Parent { protected String text; public Child(){ System.out.println("Using the default constructor of Child class.."); } public Child(int i,int j,String t){ super(i,j); System.out.println("Using the integer constructor of Child class.."); this.text=t; } public void showMessage(){ showMessage("Java programming is very interesting!!"); System.out.println("A message from Child class.."); System.out.println("Message : "+text); } } class InherianceStudy { public static void main(String args[]){ System.out.println("Creating Parent objects..."); System.out.println("_________________________________________________\n"); Parent p1=new Parent(); Parent p2=new Parent(2,5); System.out.println("\nCreating Child objects..."); System.out.println("_________________________________________________\n"); Child c1=new Child(); Child c2=new Child(3,-1,"Java is owned by Sun Microsystems"); System.out.println("\nShowing messages.."); System.out.println("_________________________________________________\n"); c2.showMessage(); } }Output:
ඉහත ජාවා කේතය දී ඇති ප්රතිඵලය සමග සැසඳීමෙන් ඔබට Inheritance සංකල්පය ගැන හොඳ අවබෝධයක් ලබා ගැනීමට පුලුවන්. Sub class එකකින් object එකක් සාදන සෑම විටම එහි super class එකත් construct වීම සිදුවන බව දැන් ඔබට වටහා ගත හැකිවිය යුතුයි.
මෙම පාඩමේ උදාහරණ download කරගැනීමට පහත Link එක භාවිතා කරන්න.
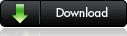
cube example eke,
ReplyDeleteconstructor1 this.color wenuwata color kiya dammama output1 enne ne,
eth, anik this.color wenuwata color dammata output1ta awlak nene...
හොද වැඩක් දිගටම කරගෙන යන්න.
ReplyDeleteමට අහම්බෙන් ලැබුනේ මම සුභ පතනවා.. ගොඩක් වැදගත් වෙන දෙවල් තියෙනවා ස්තුතියි යාලුවා