අපි මුලින්ම සරල උදාහරණයකින් පටන්ගනිමු. මෙහිදී ඉදිරිපත් කර ඇති කේතයන් ඔබ ඉගෙනගත් OOP concept සමග ගලපමින් අවබෝධ කරගැනීමට උත්සාහ කරන්න.
Exercise1
/** *Author : Kanishka Dilshan *Blog : http://javaxclass.blogspot.com *Purpose: Demonstrate some applications of OOP */ class Car { private String model,color; private int enginePower,speed; public Car(String model,String color,int enginePower){ this.model=model; this.color=color; this.enginePower=enginePower; speed=0; } public void accelerate(){ //let's assume the maximum speed is 320 if(speed < 320){ speed+=10; //increment speed by 10 } } public void applyBreaks(){ if(speed > 10){ speed-=10; //decrement speed by 10 } } public void changeColor(String NewColor){ this.color=NewColor; } public void showDetails(){ System.out.println("----------------------------------"); System.out.println("Car Model : " + model); System.out.println("Engine Power : " + enginePower); System.out.println("Color : " + color); System.out.println("Current Speed: " + speed); System.out.println("----------------------------------"); } public String getModel(){ return model; } } public class Exercise1{ public static void main(String args[]){ //create a new Car object Car c1=new Car("Audi A4","Blue",2000); //accelerate the car for(int i=0;i < 120;i++){ c1.accelerate(); } //show details of the car c1.showDetails(); //apply breaks on the car(3 times) c1.applyBreaks(); c1.applyBreaks(); c1.applyBreaks(); //change color c1.changeColor("Black"); //show details of the car again c1.showDetails(); //create another car object Car c2=new Car("Mazda 3 Sedan","Red",1800); //....................... } }Output :
දැන් අපි ඉහත ලියන ලද ජාවා වැඩසටහන විස්තරාත්මක වශයෙන් සාකච්ඡා කරමු.
Line 07 මගින් Car class එකේ ආරම්භය සනිටුහන් කෙරෙනවා. එහි සියලුම class variables වලට යොදා ඇත්තේ private modifier එක බව ඔබට පෙනෙනවා ඇති.(line 8,line 9). අපි access modifiers ගැන සාකච්ඡා කරද්දී ඔබට මතක ඇති මම එහිදී සඳහන් කලා instance variables,private ලෙසත් methods , public ලෙසත් යෙදීම හොඳ වස්තු පාදක ක්රමලේඛනයක ලක්ෂණයක් බව.
ඉන්පසුව ඇත්තේ constructor එකයි (line 11) එහිදී අපි speed එක 0 ලෙසත් අනෙකුත් class variables සඳහා constructor එකෙන් ලබාගන්නා parameters ද ආදේශ කර තිබෙනවා. මෙහිදී constructor එකේ parameter එකක් ලෙස speed එක ගෙන නැහැ. නමුත් constructor එක තුලදී speed variable එක සුදුසු value එකක් යොදා initialize කර තිබෙනවා.
ඉන්පසු ඇත්තේ methods ය. අපි methods පාඩමේදී ලබාගත් දැණුම භාවිතයෙන් ඔබට මෙය පහසුවෙන් අවබෝධ කරගත හැකිවිය යුතුය.
Car class එක ලියා අවසන්වූ පසු main method එක අයත් Exercise1 class එක ආරම්භවේ. මෙහිදී අප සකස්කරගත් Car class එක භාවිතා කර object සාදා ඒවා භාවිතා කිරීම සිදු කෙරේ. ජාවා වැඩසටහන තුල යොදා ඇති comments වලින්ද එහිදී සිදුවන්නේ කුමක්ද යන්න තේරුම් ගැනීමට හැක.
Exercise2
/** *Class Name : Excerice2java *Author : Kanishka Dilshan *Blog : http://javaxclass.blogspot.com *Purpose : Demonstrate some applications of OOP */ class Line { private int x1,y1,x2,y2; public Line(int x1,int y1,int x2,int y2){ this.x1=x1; this.y1=y1; this.x2=x2; this.y2=y2; } public double getLength(){ double length=Math.sqrt(Math.pow((x1-x2),2)+Math.pow((y1-y2),2)); return length; } } public class Exercise2{ public static void main(String args[]){ Line line1=new Line(-3,6,10,8); double len=line1.getLength(); System.out.println(len); } }අපි ඊලඟ පාඩමෙන් මීට වඩා සංකීර්ණ උදාහරණ කිහිපයක් බලමු.
මෙම පාඩමේ ඉදිරිපත් කර ඇති මූලාශ්ර කේත භාගත කරගැනීමට පහත link එක භාවිතා කරන්න.
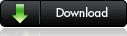
0 comments:
Post a Comment